728x90
반응형
경로를 입력받아 폴더인지 파일인지 확인하기
C++ 17로 윈도우에서 프로그램을 만들면서 폴더와 파일을 구분해야하는 경우가 생겨 아래 코드를 찾게되었다.
찾은 코드는 테스트 시 작동에는 문제가 없었지만 문자열을 LPCWSTR (WCHAR *)로 받아야 한다는 사소한 단점이 있었다. 혹시 String 클래스를 사용해 입력받은 경로가 폴더인지 파일인지 구분할 수 있는 방법이 있는지도 찾아봤지만, 검색을 해봐도 찾지 못했다..
int isFolder(LPCWSTR path) {
/*
args:
LPCWSTR fileName : 파일의 full path
return:
int code : 폴더는 1, 파일은 0, 에러는 -1
summary:
폴더인지 파일인지 구분
*/
WIN32_FIND_DATA wInfo;
HANDLE hInfo = ::FindFirstFile(path, &wInfo);
::FindClose(hInfo);
if (hInfo != INVALID_HANDLE_VALUE)
{
if (wInfo.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY)
{
return 1;
}
return 0;
}
return -1;
}
출처 : https://jangjy.tistory.com/388
int main() {
cout << isFolder(L"C:\\Users\\user\\Downloads") << "\n";
return 0;
}
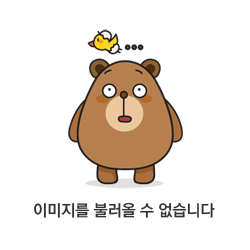
String to LPCWSTR
위 내용에서 함수를 찾긴했지만 입력받는 방식이 달라 사용이 어려웠다.
작성중인 프로그램에서는 문자열을 String 클래스를 사용하여 처리하기 때문에 변환이 필요했는데, 구글링을 통해 아래 코드를 알게 되었다.
std::wstring s2ws(const std::string& s)
{
int len;
int slength = (int)s.length() + 1;
len = MultiByteToWideChar(CP_ACP, 0, s.c_str(), slength, 0, 0);
wchar_t* buf = new wchar_t[len];
MultiByteToWideChar(CP_ACP, 0, s.c_str(), slength, buf, len);
std::wstring r(buf);
delete[] buf;
return r;
}
출처 : https://wiserloner.tistory.com/316
위의 코드를 아래처럼 사용하면 변환된 결과를 얻을 수 있다.
int main() {
std::wstring stemp = s2ws("C:\\Users\\user\\Downloads");
LPCWSTR result = stemp.c_str();
wprintf(result);
return 0;
}
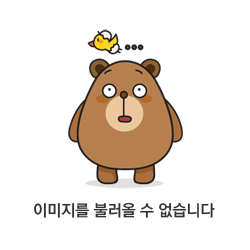
String으로 폴더 여부 확인하기
이제 isFolder() 함수가 String을 사용해 경로를 확인할 수 있도록 수정해보자.
#include <windows.h>
#include <iostream>
#include <string>
#include <fstream>
#include <filesystem>
#include <vector>
#include <map>
using namespace std;
wstring s2ws(const string& s) {
int slength = (int)s.length() + 1;
int len = MultiByteToWideChar(CP_ACP, 0, s.c_str(), slength, 0, 0);
wchar_t* buf = new wchar_t[len];
MultiByteToWideChar(CP_ACP, 0, s.c_str(), slength, buf, len);
wstring r(buf);
delete[] buf;
return r;
}
int isFolder(string path) {
/*
args:
LPCWSTR fileName : 파일의 full path
return:
int code : 폴더는 1, 파일은 0, 에러는 -1
summary:
폴더인지 파일인지 구분
*/
WIN32_FIND_DATA wInfo;
HANDLE hInfo = ::FindFirstFile(s2ws(path).c_str(), &wInfo);
::FindClose(hInfo);
if (hInfo != INVALID_HANDLE_VALUE){
if (wInfo.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY){
return 1;
}
return 0;
}
return -1;
}
int main() {
cout << isFolder("C:\\Users\\user\\Downloads") << "\n";
return 0;
}
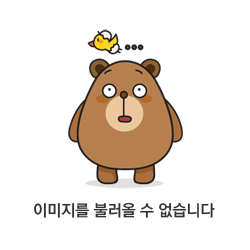
728x90
반응형
'프로그래밍 > Windows' 카테고리의 다른 글
[Windows Programming] 모니터 제어 명령 세트(MCCS)를 활용한 모니터 제어 프로그램 (0) | 2022.08.07 |
---|---|
[Win32 API] GetCurrentDirectory : 현재 디렉토리 위치 구하기 (0) | 2022.06.01 |
[Win32 API] PathFileExists : 파일 존재 여부 확인하기 (0) | 2022.06.01 |
[Windows] CallBack 함수 (0) | 2022.05.07 |
[WPF] YouTube Player (0) | 2022.03.10 |
[WPF] OpenCvSharp4로 윈도우 안에 카메라 영상 그리기 (0) | 2022.02.02 |